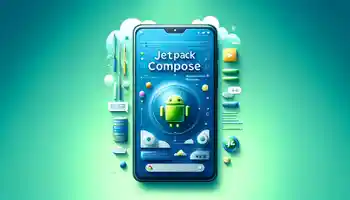
In iOS app development, changing fonts manually through storyboards for each UI element can be time-consuming and prone to errors, especially when updates are needed across the app. This manual process becomes inefficient as projects grow.
The Custom Font SDK solves this by allowing developers to manage and update fonts programmatically. With this SDK, you can easily load custom fonts without manually updating each element in the storyboard, saving time and reducing errors.
Get the SDK: Download the Custom Font SDK.
Use the following code snippet to load your custom fonts into the SDK:
CustomFont.FontStyle.storeFontNames(ultralight: "font name" thin:"font name", light: "font name", regular: "font name", medium: "font name", semiBold: "font name", bold: "font name", heavy: "font name", black: "font name")
This method will store your custom font names in the SDK and allow you to access them throughout the app.
import CustomFont
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
customFont()
return true
}
// Load this method on didFinishLaunchingWithOptions
func customFont(){
CustomFont.FontStyle.storeFontNames(ultralight: "font name" thin:"font name", light: "font name", regular: "font name", medium: "font name", semiBold: "font name", bold: "font name", heavy: "font name", black: "font name")
UIFont.overrideInitialize()
}
}
Here you can store your font style names to sdk.