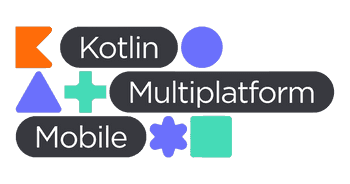
With Android Jetpack Compose, the way we build UI in Android has completely transformed. Unlike the traditional approach where XML files handle the UI while Kotlin or Java handles the logic, Compose allows you to build both the UI and functionality using a single language Kotlin.
Gone are the days of switching between XML layouts and Java/Kotlin code. In Compose, everything happens in one place, making development faster and more efficient. So why is it called "Compose"?
In the old Android UI system, every visual element you added to your screen had to inherit from a base class View
. For example, if you wanted to show text, you'd use TextView
, which inherits from View
. Similarly, interactive components like EditText
also inherit from TextView
and ultimately from View
.
Compose changes this paradigm completely by using a composition-based system instead of inheritance. In Compose, your UI elements don’t inherit from a base class like View. Instead, you "compose" your UI by combining different smaller elements (composables) together. This flexibility allows you to easily build complex UIs by reusing and combining smaller building blocks.
For example, in Compose, creating a text input field or a button is as simple as writing a few lines of Kotlin code, all without the need for XML:
Text("Hello, World!")
Button(onClick = { /* Do something */ }) {
Text("Click Me")
}
@Composable
fun SimpleUI() {
Column(modifier = Modifier.fillMaxSize(), horizontalAlignment = Alignment.CenterHorizontally) {
Text(text = "Hello, Compose!")
Button(onClick = { /* Handle click */ }) {
Text(text = "Click Me")
}
}
@Composable
fun Greeting() {
var name by remember { mutableStateOf("World") }
Column(modifier = Modifier.fillMaxSize(), horizontalAlignment = Alignment.CenterHorizontally) {
Text(text = "Hello, $name!")
Button(onClick = { name = "Compose" }) {
Text("Change Name")
}
}
}
In this code, we use remember
to create a name
state variable initialized to "World." This keeps the value consistent across recompositions. The Text
composable displays the current name
, and when the button is clicked, the onClick
lambda updates it to "Compose," automatically reflecting the change in the UI.
padding
, border
, and background
color. @Composable
fun CustomButton(text: String, onClick: () -> Unit) {
Button(
onClick = onClick,
modifier = Modifier
.padding(16.dp)
.border(2.dp, Color.Blue, shape = RoundedCornerShape(8.dp))
.background(Color.Yellow)
) {
Text(text = text)
}
}
@Composable
fun CustomUI() {
Column(modifier = Modifier.fillMaxSize(), horizontalAlignment = Alignment.CenterHorizontally) {
CustomButton(text = "Custom Button") {
// Handle button click
}
}
}
To conclude, Jetpack Compose is more than just a new way to build UIs—it’s a game changer for Android development. By moving away from XML layouts and embracing a composition model, Compose makes the development process easier and more flexible, allowing developers to build dynamic UIs with less code. Its modern, declarative approach is set to become the standard for Android apps. There's much more to explore! In upcoming posts, we'll cover advanced topics like effective state management, and how to migrate existing projects to Jetpack Compose. Stay tuned for Part 2!