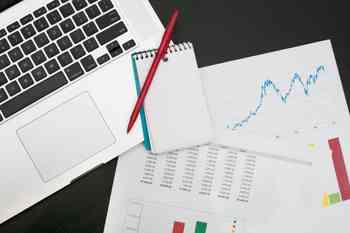
Automation scripts play a vital role in enhancing the efficiency of testing processes. However, as projects grow in complexity, poorly written scripts can become a maintenance nightmare. Following best practices ensures that your automation scripts are robust, scalable, and easy to maintain. Here’s a guide to writing maintainable automation scripts:
Organize your scripts to reflect a clean and consistent structure. Use a framework or design pattern such as Page Object Model (POM) for UI automation or a modular approach for API and load testing. Group reusable components like utilities, configuration files, and test data separately to avoid duplication.
Give meaningful names to variables, functions, and files. A good naming convention enhances code readability and helps team members understand the script’s purpose without digging through its logic. For example:
login_with_valid_credentials
is better than test1
.validate_token_expiry
is better than check1
.Avoid hardcoding values. Use configuration files, environment variables, or test data files to manage dynamic data like URLs, credentials, and API keys. Parameterization ensures that scripts are reusable across environments and scenarios.
# Example in Python
BASE_URL = os.getenv('BASE_URL')
def test_endpoint():
response = requests.get(f"{BASE_URL}/api/v1/resource")
assert response.status_code == 200
Break your script into smaller, reusable modules. Modular scripts allow testers to update or fix issues in one place without impacting the entire codebase. A modular design enhances maintainability, readability, and scalability.
# Example: Modular Design in Python
class AuthModule:
def login(self, username, password):
# Login logic
pass
def logout(self):
# Logout logic
pass
class DataValidationModule:
def validate_response(self, response):
# Validation logic
pass
# Utility function to handle API requests
def make_api_request(endpoint, method="GET", data=None):
response = requests.request(method, endpoint, json=data)
response.raise_for_status()
return response
Think of it like building blocks: instead of creating one big block (a class with multiple inherited layers), you use multiple smaller blocks that can work together. This approach makes it easier to update or replace parts of your code without breaking everything else.
A modular design not only simplifies debugging and maintenance but also ensures that changes in one part of the system have minimal impact on others.
Include comments and docstrings to explain the purpose of scripts and functions. Comprehensive documentation helps onboard new team members quickly and simplifies debugging.
# Function to verify login functionality
# Parameters: username (str), password (str)
def login(username, password):
# Your login logic here
Write scripts to handle expected and unexpected errors gracefully. Use try-except blocks and log meaningful error messages to diagnose issues effectively.
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error occurred: {e}")
Make assertions meaningful and ensure they verify critical parts of the functionality. Overusing assertions or placing them incorrectly can make debugging difficult.
assert response.status_code == 200, "Expected status code 200, got {response.status_code}"
assert "token" in response.json(), "Token not found in response"
Use a version control system like Git to manage your scripts. Commit changes frequently with meaningful messages and use branching strategies to work collaboratively.
Integrate your automation scripts into CI/CD pipelines to run them automatically on code changes. This ensures early detection of issues and maintains quality.
Adopt the "Don't Repeat Yourself" (DRY) principle. Avoid duplicating code by using reusable functions or libraries. Centralize common logic to simplify updates and fixes.
Schedule periodic reviews to remove obsolete tests, improve logic, and incorporate new best practices. Refactoring ensures scripts remain efficient and aligned with project goals.
Writing maintainable automation scripts requires effort, but the long-term benefits of scalability and reduced technical debt outweigh the initial investment. By following these best practices, teams can ensure that their automation efforts remain efficient and adaptable to changing requirements. Remember, the key to maintainable automation is not just about writing code but writing code that your future self and team members will thank you for!